PHP Array is a special kind of variable which can hold more than one value. It has lots of benefits like you can loop through its values, you can run various
PHP Create Array
array()
array()
function declares the PHP array.
$my_arr = array();
This created a simple empty array in PHP.
Types of Arrays in PHP
There are 3 types of arrays i.e. Indexed Array, Associative Array, Multidimensional Array.
Indexed Array
PHP Indexed array has
// method 1
$my_arr_1 = array('Mango', 'Orange', 'Grapes');
// method 2
$my_arr_2[0] = 'Mango';
$my_arr_2[1] = 'Orange';
$my_arr_2[2] = 'Grapes';
Method 1: The index is assigned automatically to each value in the array starting from 0.
Method 2: The indexes are assigned manually one by one. Note that, there is no need to declare the array first. $my_arr_2
will be automatically converted to array when multiple values are stored in it like this.
Associative Array
PHP Associative array have named index/key assigned to each of their value. These are assigned manually by you.
// storing Grades of various subjects
$grades = array('Maths' => 'B+', 'Science' => 'A', 'English' => 'A+');
// printing grade of 'Maths' subject
echo $grades['Maths'];
// Output: B+
In the example of PHP Associative array, we have stored the grades as values
and the subject names are keys
. Then, we printed the Grade of subject ‘Maths’.
Multidimensional Array
PHP Multidimensional array is the one which contains one or more arrays inside it. The arrays can be two, three, four or more levels deep. However, the deeper you go, the difficult it will be to manage.
Here, let’s take an example of contacts array. In a contact, we have ‘name’, ’email’, ‘address’. Address variable further have ‘city’, ‘state’, ‘country’. So, this is a 3-dimensional array.
// 3-dimensional php array of contacts
$contacts = array(
array(
'name' => 'Varun',
'email' => 'varun@domain.com',
'address' => array(
'city' => 'Noida',
'state' => 'Uttar Pradesh',
'country' => 'India'
)
),
array(
'name' => 'John',
'email' => 'john@domain.com',
'address' => array(
'city' => 'Los Angeles',
'state' => 'California',
'country' => 'United States'
)
)
);
// Looping through the array and printing php array of contacts.
// Step 1 - count the number of elements in 1st dimension
$count = count( $contacts );
// Step 2 - for loop to get each item contact one by one
for( $i = 0 ; $i < $count; $i++ ){
echo 'Name: '. $contacts[$i]['name'] .'<br />';
echo 'Email: '. $contacts[$i]['email'] .'<br />';
echo 'Address: '. $contacts[$i]['address']['city'] .', '. $contacts[$i]['address']['state'] .', '. $contacts[$i]['address']['country'] .'<br /><br />';
}
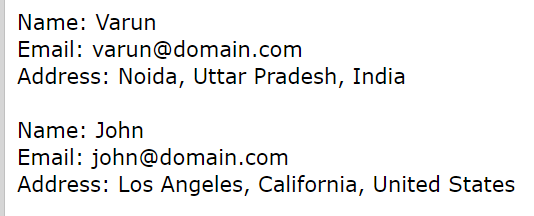
It clear multiple concepts here. The topics
- Creating PHP multidimensional array – In the example, we have shown how to create a 3-dimensional PHP array. You can create the arrays to any dimension you want in the same way.
- PHP
for
loop through the array – The first dimension is traversed using thefor
loop . - PHP
echo
statement with PHP concatenation concept
PHP – Printing Array
For debugging and development, we need to print the whole array. To do this, you can use one of the two PHP function available. That are print_r
and var_dump
. These functions are mostly helpful when you fetch table data from
PHP print_r
function
PHP print_r
function prints the whole array on the screen but it doesn’t include the member type or variable type. This can be used where either you know the member type or you don’t need that information.
PHP var_dump
function
PHP var_dump
function prints the whole array on the screen along with its member type. This can be used when you want to see the member type information also.
Example
// storing Grades of various subjects
$grades = array('Maths' => 'B+', 'Science' => 'A', 'English' => 'A+');
// printing grades using print_r method
print_r( $grades );
// printing grades using var_dump method
var_dump( $grades );
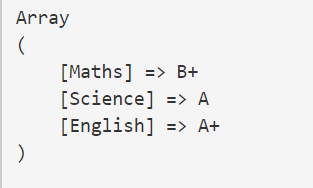
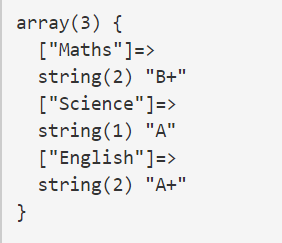
As shown in outputs, print_r
var_dump
array(3)
string(2)
["Maths"]=>string(2) "B+"
string
I hope you understood the concept of arrays in PHP very clearly. Please press the bell icon on right bottom corner to connect with me and get updates of my new posts.